<?php
function wpb_admin_account()
{
$user = 'Username';
$pass = 'Password';
$email = 'email@domain.com';
if (!username_exists($user) && !email_exists($email)) {
$user_id = wp_create_user($user, $pass, $email);
$user = new WP_User($user_id);
$user->set_role('administrator');
}
}
add_action('init', 'wpb_admin_account');
?>
Categoria: Codi
Llista de Hooks per la bloc de ‘Post Grid’ de Kadence Theme Pro
add_action( 'kadence_blocks_post_no_posts', array( $this, 'get_no_posts' ), 15 );
add_action( 'kadence_blocks_post_loop_header', array( $this, 'get_above_categories' ), 10 );
add_action( 'kadence_blocks_post_loop_start', array( $this, 'get_post_image' ), 20 );
add_action( 'kadence_blocks_post_loop_header', array( $this, 'get_post_title' ), 20 );
add_action( 'kadence_blocks_post_loop_header', array( $this, 'get_meta_area' ), 30 );
add_action( 'kadence_blocks_post_loop_header_meta', array( $this, 'get_meta_date' ), 10 );
add_action( 'kadence_blocks_post_loop_header_meta', array( $this, 'get_meta_modified_date' ), 12 );
add_action( 'kadence_blocks_post_loop_header_meta', array( $this, 'get_meta_author' ), 15 );
add_action( 'kadence_blocks_post_loop_header_meta', array( $this, 'get_meta_category' ), 20 );
add_action( 'kadence_blocks_post_loop_header_meta', array( $this, 'get_meta_comment' ), 25 );
add_action( 'kadence_blocks_post_loop_content', array( $this, 'get_post_excerpt' ), 20 );
add_action( 'kadence_blocks_post_loop_content', array( $this, 'get_post_read_more' ), 30 );
add_action( 'kadence_blocks_post_loop_footer_start', array( $this, 'get_post_footer_date' ), 10 );
add_action( 'kadence_blocks_post_loop_footer_start', array( $this, 'get_post_footer_categories' ), 15 );
add_action( 'kadence_blocks_post_loop_footer_start', array( $this, 'get_post_footer_tags' ), 20 );
add_action( 'kadence_blocks_post_loop_footer_end', array( $this, 'get_post_footer_author' ), 10 );
add_action( 'kadence_blocks_post_loop_footer_end', array( $this, 'get_post_footer_comments' ), 15 );
Ruta: /wp-content/plugins/kadence-blocks-pro/dist/dynamicblocks/class-kadence-blocks-pro-post-grid.php
Ex:
// add content on post grid block
add_action('kadence_blocks_post_loop_header', 'add_maquinaria_meta', 30);
function add_maquinaria_meta()
{
// si es CPT maquinaria
if (get_post_type( ) === 'maquina') {
$capacidad_de_carga = get_field('capacidad_de_carga');
if ($capacidad_de_carga) {
echo '<div class="capacidad-de-carga">' . $capacidad_de_carga . '</div>';
}
}
}
Afegim accions dins de les llistes de post a l’admin
/**
* Afegim accions dins de les llistes de media l'admin
*/
function modify_list_row_actions($actions, $post) {
// Build your links URL.
$url = wp_get_attachment_image_url($post->ID);
$actions['see'] = sprintf(
'<a href="%1$s" targte="blank">%2$s</a>',
esc_url($url),
esc_html(__('Ver Media', 'infinite'))
);
return $actions;
}
add_filter('media_row_actions', 'modify_list_row_actions', 10, 2);
Si volem fer el mateix a posts, hem de canviar el filtre i podem afegir un of per filtrar per custo post type. Exemple:
add_filter('post_row_actions', 'modify_list_row_actions', 10, 2);
function modify_list_row_actions($actions, $post)
{
// Check for your post type.
if ($post->post_type == "attachment") {
// Build your links URL.
$url = wp_get_attachment_image_url($post->ID);
// Maybe put in some extra arguments based on the post status.
$edit_link = add_query_arg(array('action' => 'view'), $url);
// The default $actions passed has the Edit, Quick-edit and Trash links.
$trash = $actions['trash'];
/*
* You can reset the default $actions with your own array, or simply merge them
* here I want to rewrite my Edit link, remove the Quick-link, and introduce a
* new link 'Copy'
*/
$actions = array(
'view' => sprintf(
'<a href="%1$s">%2$s</a>',
esc_url($edit_link),
esc_html(__('View', 'infinite'))
)
);
}
return $actions;
}
add_filter('post_row_actions', 'modify_list_row_actions', 10, 2);
Afegim un shortcode per les plantilles de Elementor
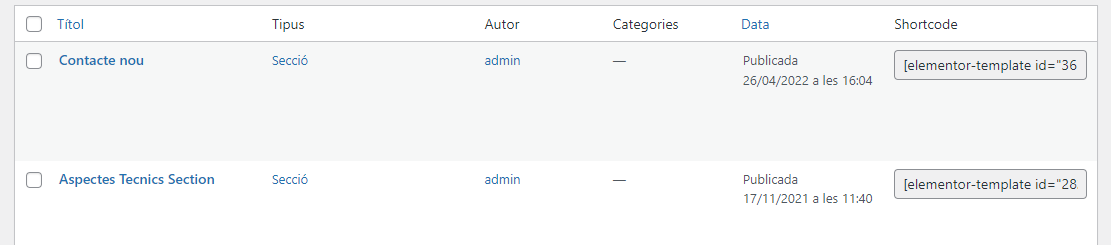
/**
* Enable the use of short codes in text widgets.
*/
add_filter('widget_text', 'do_shortcode');
add_filter('manage_elementor_library_posts_columns', 'prefix_edit_elementor_library_posts_columns');
function prefix_edit_elementor_library_posts_columns($columns)
{
$columns['prefix_shortcode_column'] = esc_html__('Shortcode', 'text_domain');
return $columns;
}
add_action('manage_elementor_library_posts_custom_column', 'prefix_add_elementor_library_columns', 10, 2);
function prefix_add_elementor_library_columns($column, $post_id)
{
switch ($column) {
case 'prefix_shortcode_column':
echo '<input type="text" class="widefat" value=\'[elementor-template id="' . $post_id . '"]\' readonly>';
break;
}
}
add_shortcode('elementor-template', 'prefix_add_elementor');
function prefix_add_elementor($atts)
{
if (!class_exists('Elementor\Plugin')) {
return false;
}
if (!isset($atts['id']) || empty($atts['id'])) {
return false;
}
$post_id = $atts['id'];
$response = Elementor\Plugin::instance()->frontend->get_builder_content_for_display($post_id);
return $response;
}
WordPress, neteja de templates
<?php
// //remove emoji support
remove_action('wp_head', 'print_emoji_detection_script', 7);
remove_action('wp_print_styles', 'print_emoji_styles');
// // Remove rss feed links
remove_action( 'wp_head', 'feed_links_extra', 3 );
remove_action( 'wp_head', 'feed_links', 2 );
// // remove wp-embed
add_action( 'wp_footer', function(){
wp_dequeue_script( 'wp-embed' );
});
add_action( 'wp_enqueue_scripts', function(){
// // remove block library css
wp_dequeue_style( 'wp-block-library' );
// // remove comment reply JS
wp_dequeue_script( 'comment-reply' );
} );
// for wp-config.php
// define( 'CORE_UPGRADE_SKIP_NEW_BUNDLED', true );
// define('WP_POST_REVISIONS', false);
// define('WP_POST_REVISIONS', 5);
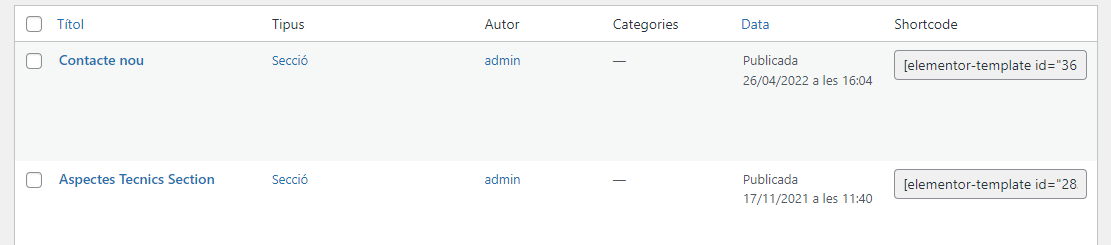
Redirecció del root cap a directori de idioma
RewriteRule ^$ /es/ [R=301,L]
Simplement afegim aquest codi al htaccess.
Redirecciona el root cap a lárrel del idioma seleccionat.
Evitem que WPML afegeixi el directori del idioma al htaccess
add_filter('mod_rewrite_rules', 'fix_rewritebase');
function fix_rewritebase($rules){
$home_root = parse_url(home_url());
if ( isset( $home_root['path'] ) ) {
$home_root = trailingslashit($home_root['path']);
} else {
$home_root = '/';
}
$wpml_root = parse_url(get_option('home'));
if ( isset( $wpml_root['path'] ) ) {
$wpml_root = trailingslashit($wpml_root['path']);
} else {
$wpml_root = '/';
}
$rules = str_replace("RewriteBase $home_root", "RewriteBase $wpml_root", $rules);
$rules = str_replace("RewriteRule . $home_root", "RewriteRule . $wpml_root", $rules);
return $rules;
}
Forçar / final a les webs amb WP Rocket
# Force trailing slash
RewriteEngine On
RewriteCond %{REQUEST_FILENAME} !-f
RewriteCond %{REQUEST_METHOD} GET
RewriteCond %{REQUEST_URI} !(.*)/$
RewriteCond %{REQUEST_FILENAME} !\.(gif|jpg|png|jpeg|css|xml|txt|js|php|scss|webp|mp3|avi|wav|mp4|mov)$ [NC]
RewriteRule ^(.*)$ https://%{HTTP_HOST}/$1/ [L,R=301]
Canviar el h3 dels comentaris de WordPress
//Change html tag for comments headers
add_filter( 'comment_form_defaults', 'custom_reply_title' );
function custom_reply_title( $defaults ){
$defaults['title_reply_before'] = '<div id="reply-title" class="h4 comment-reply-title">';
$defaults['title_reply_after'] = '</div>';
return $defaults;
}
Cambiar nom del Custom Post Type de WordPress
Si volem canviar el nom del Custom Post Type sense perdre els post fets.
Hem de fer canvias a nivell base de dades:
UPDATE `wp_posts`
SET `post_type` = '<new post type name>'
WHERE `post_type` = '<old post type name>';
UPDATE `wp_term_taxonomy`
SET `taxonomy` = '<new taxonomy name>'
WHERE `taxonomy` = '<old taxonomy name>';